I'm sorry if this has been answered before, it's not the easiest thing to google.
I'm adding sound to my 48k game and I can hit the beeper and get sound from it, but I can't find anywhere explaining or giving sound 'samples'.
Eg I've got an array of '0,1,1,0,0,0,0,1,1,1,0,1' etc. and I get random sound from it by messing with these numbers but is there anywhere that I can find someone's array to get me, for example, a jump sound, a collect style sound etc?
I can't sit and try random sets of numbers searching for the right sound, I've been messing with it for days, and I've not managed to get anything as good as even Chucky egg.
This is for a very fast (~25-50fps) scrolling platform game, I'm actually trying to make the fastest scrolling game ever made on the 48k with parallax etc. hitting the sound chip does not slow the game down as much as I expected, so I can throw in quite a lot of beeper sound, maybe even simple music.
Thanks!
Here is a pic to show the game is real, I have a demo too if anyone wants to see it (the name at the top isn't final, it just started as a Mario clone).
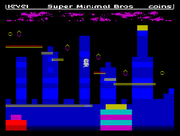