I'm going to have 2 maps, one for each 4bit shift attribute.
So all the ASM has to do is read the section of the map to the attribute screen and like magic it will 'scroll'.
It's been a long time since I wrote anything in ASM, and I want to modify this to deal with it as it'll be too slow in C:
org 0x5ccb ; start of code
LD A,1
LD B,8 ; Number of times to loop (8 bits)
scroll
ld de,0x4000 ; DE = screen
LD B,A
LOOP:
inc DE
DJNZ LOOP ; Decrease B, and loop A times
inc a
Start
ld hl,ImgData ; HL = image data
ld bc,0x1aff ; 6144 bytes bitmap, 768 bytes attributes ldir counts this down until 0
ldir ; Repeats LDI (LD (DE),(HL), then increments DE, HL, and decrements BC) until BC=0. Note that if BC=0 before this instruction is called, it will loop around until BC=0 again.
;Loop
jp scroll ; infinite loop
ImgData ; data file
incbin "themap.bin"
org 0xff57
defb 00h ; end of ROM
Is this a good starting point? Is the way I'm increasing DE wrong? I've done a little loop... I need to unnderstand how to put C variables in to ASM code really.
Here is a picture of the layout of the screen to get an idea what I'm doing, all the scrolling is done by manipulating the attribute later. The sprites are then drawn on top.
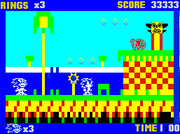