I'd love to hear your list of "any of those other isometric games" that use a regular grid of fixed-size blocks. Have you got a single example beyond Zombie Zombie?
It's not even close to how voxel-based games on PCs work.
Once again: traverse the map to figure out which surfaces are visible. Because it's regular, and all cubes exactly fill a block.Joefish wrote: ↑Mon May 17, 2021 1:14 pmbut the Spectrum doesn't have the processing power to work like that. Any one block might only be partly obscuring another block. So do you divide all your blocks up into 8 triangles and check against all 8 x 1/8s of the blocks that may be behind it? Or is it quicker just to draw them all over the top of each other?
So, no, at no point are you going to work forwards from cubes.
It looks like I last implemented it 16 years ago, here's the entire casting function:
Code: Select all
#define GetByte(x, y) ( ((x) < -64) || ((y) < -64) || ((x) > 63) || ((y) > 63) ) ? 0 : map[((x+64) << 7) | (y+64)]
void LaunchRay(int mapx, int mapy, int x, int y)
{
int mask = 0x20;
int byte1, byte2, byte3, byte4;
int leftempty = 1, rightempty = 1;
byte4 = GetByte(mapx, mapy);
while(mask && (leftempty || rightempty))
{
byte1 = byte4;
byte2 = GetByte(mapx + LeftX, mapy + LeftY); //left one
byte3 = GetByte(mapx + DownX, mapy + DownY); //up one
mapx += LeftX + DownX;
mapy += LeftY + DownY;
byte4 = GetByte(mapx, mapy); //left & up one
if(leftempty)
{
if(byte1&mask)
{
draw_sprite(back, ul, x, y);
leftempty = 0;
}
else
{
if(byte2&mask)
{
draw_sprite(back, mr, x, y);
leftempty = 0;
}
else
if(byte4&mask)
{
draw_sprite(back, bl, x, y);
leftempty = 0;
}
}
}
if(rightempty)
{
if(byte1&mask)
{
draw_sprite(back, ur, x+left->w, y);
rightempty = 0;
}
else
{
if(byte3&mask)
{
draw_sprite(back, ml, x+left->w, y);
rightempty = 0;
}
else
if(byte4&mask)
{
draw_sprite(back, br, x+left->w, y);
rightempty = 0;
}
}
}
mask >>= 1;
}
if(leftempty && byte4)
draw_sprite(back, shadowl, x, y);
if(rightempty && byte4)
draw_sprite(back, shadowr, x+left->w, y);
}
That's casting into exactly the Ant Attack data format, by the way. Here's some sample output:
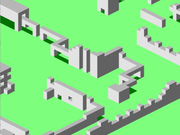
`draw_sprite` is an Allegro 4.x call, because as I said this code is 16 years old. It doesn't actually need a 'sprite' routine, they're always character-square aligned and never overlap; even with my inclusion of shadows there's a grand total of five of them. Get rid of the shadows and it'd be three.
EDIT: oh, and this is also exactly how Snake, Rattle & Roll on the NES works per its tile set, except that Rare have gone one step further and allowed for split blocks that have a different graphic for their left and right parts.
So, no, despite your claim that the algorithm above is intractable for some unspecified reason, Rare didn't somehow manage to draw from back to front, to a constructed bitmap, fast enough to scroll, on a NES.