Short fallout style text game (Merged from multiple topics)
Re: Short fallout style text game (Merged from multiple topics)
oh i see thanks, I was assuming that zero was in the middle, like at position 128 and -128 was in the zero position of a normal byte.
So I thought that +128 was where 255 would normally be.
I see how it is now lol
one slight problem is CP because it would say 255 is bigger than zero all the time, ignoring the possibility that its really -1 and therefore smaller than zero. Unless there is a signed version of CP
http://z80-heaven.wikidot.com/instructions-set:cp
oh it has signed and unsigned results.
SIGNED
If A < N, then S and P/V are different.
A >= N, then S and P/V are the same.
OKAY now i see lol. That was hard to understand at first.
So I thought that +128 was where 255 would normally be.
I see how it is now lol
one slight problem is CP because it would say 255 is bigger than zero all the time, ignoring the possibility that its really -1 and therefore smaller than zero. Unless there is a signed version of CP
http://z80-heaven.wikidot.com/instructions-set:cp
oh it has signed and unsigned results.
SIGNED
If A < N, then S and P/V are different.
A >= N, then S and P/V are the same.
OKAY now i see lol. That was hard to understand at first.
- ParadigmShifter
- Manic Miner
- Posts: 670
- Joined: Sat Sep 09, 2023 4:55 am
Re: Short fallout style text game (Merged from multiple topics)
Yeah that's where it gets gnarly CP doesn't know anything about the sign so negative vs positive number comparisons are often more hassle than they are worth.
Also CP sets flags differently to SUB if I understood the stackexchange post from earlier?Not sure about that though.
Look here for more details
http://z80-heaven.wikidot.com/instructions-set:cp
Most of the time everything will be unsigned though I think you will find.
Also CP sets flags differently to SUB if I understood the stackexchange post from earlier?Not sure about that though.
Look here for more details
http://z80-heaven.wikidot.com/instructions-set:cp
Most of the time everything will be unsigned though I think you will find.
Re: Short fallout style text game (Merged from multiple topics)
i always suspected that "H" flag was named after hentai… now i am absolutely sure it was.ParadigmShifter wrote: ↑Fri Oct 13, 2023 5:43 pm C stands for "chibi" of course which is Japanese word for tiny/small :) and NC is non-chibi (not smaller).
- ParadigmShifter
- Manic Miner
- Posts: 670
- Joined: Sat Sep 09, 2023 4:55 am
Re: Short fallout style text game (Merged from multiple topics)

english font style keyboard
created an array of pointers to rooms
each room contains 2 pointers to description strings
it worked straight away thankfully,
as i had already done a very similar thing in printing a character's name.
It's funny cos using assembly for this is like putting an f-15 plane engine in a mini metro. It's running at like 100mhz and takes up about 2k. The font takes up about 59x8 bytes, about 300.
- ParadigmShifter
- Manic Miner
- Posts: 670
- Joined: Sat Sep 09, 2023 4:55 am
Re: Short fallout style text game (Merged from multiple topics)
Use HALT to slow it down if it is too fast, and it may prevent the flickering of the text as well if you are lucky.
Ideally you want to draw everything you need immediately after the HALT and then do all the input and game logic stuff afterwards.
If you have not got anything to draw on the first frame just jump to the input and game logic bit instead of drawing.
So something like this
Ideally you want to draw everything you need immediately after the HALT and then do all the input and game logic stuff afterwards.
If you have not got anything to draw on the first frame just jump to the input and game logic bit instead of drawing.
So something like this
Code: Select all
main:
; initialisation goes here
; nothing to draw first frame probably, since it relies on game logic? if it doesn't you don't need this
jp .dogamelogic
.mainloop
; do drawing
.dogamelogic
; game logic and input etc. here
halt
jp .mainloop
Re: Short fallout style text game (Merged from multiple topics)
I think the flickering is only in the gif. But I will probably do that anyway.
I'll check to make sure though.
I could do halt before any string printing to be lazy coder. Or before setting up a new screen
I'll check to make sure though.
I could do halt before any string printing to be lazy coder. Or before setting up a new screen
Last edited by Wall_Axe on Sat Oct 28, 2023 3:24 pm, edited 1 time in total.
- ParadigmShifter
- Manic Miner
- Posts: 670
- Joined: Sat Sep 09, 2023 4:55 am
Re: Short fallout style text game (Merged from multiple topics)
You can also time stuff by changing the border colour to see how long stuff takes.
That sets the border colour to black during the mainloop and white while it is waiting for the vblank.
The border goes a bit mental if you take more than a frame to do the mainloop though. Might be worth optimising the code if it does take more than a frame, it probably shouldn't if all you are doing is drawing text and updating menu items (although the ROM calls to draw text aren't very fast).
e.g. look how slow this is
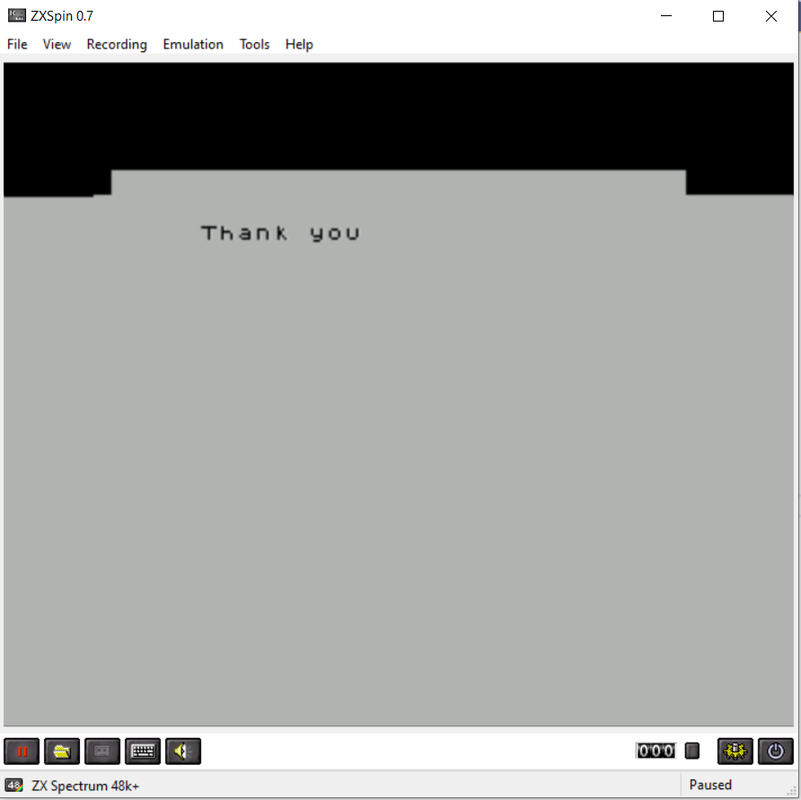
Code: Select all
.mainloop
; set border colour to black
IF TIMING
xor a
out (#FE), a
ENDIF
; stuff
; finished mainloop
; set border colour to white
IF TIMING
xor a
out (#FE), a
ENDIF
halt
jp .mainloop
The border goes a bit mental if you take more than a frame to do the mainloop though. Might be worth optimising the code if it does take more than a frame, it probably shouldn't if all you are doing is drawing text and updating menu items (although the ROM calls to draw text aren't very fast).
e.g. look how slow this is
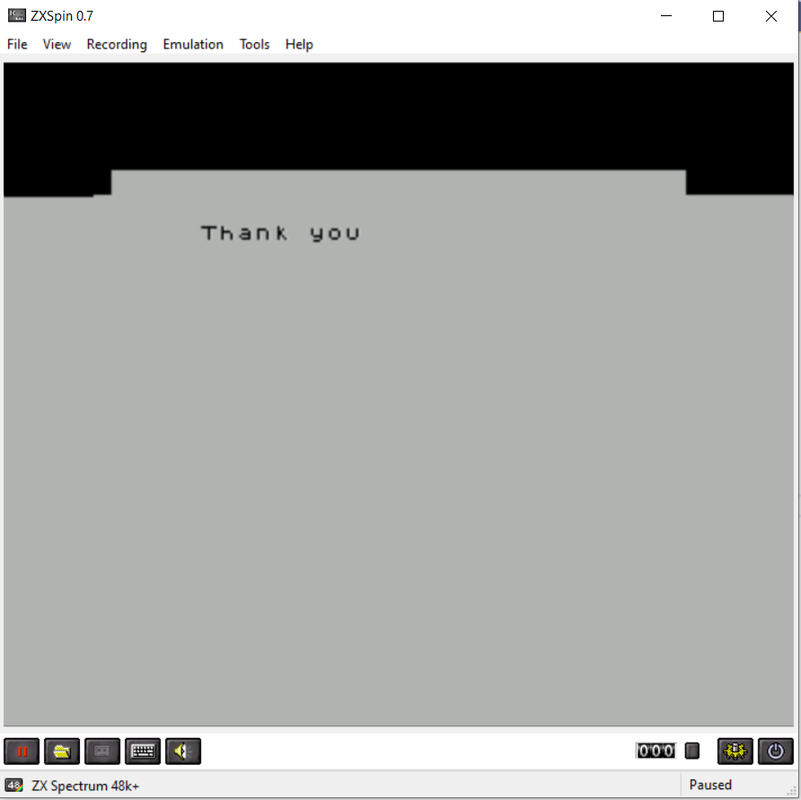
Code: Select all
ORG 32768
ld a, 2
call 5633; open channel 2
.loop
halt ; wait for vblank so we start drawing as soon as the frame starts
ld a, 0
out (#fe), a ; set the border to black
ld de, DATA1
ld bc, DATA1END-DATA1 ; length of string & control codes
call 8252
ld a, 7
out (#fe), a ; done, set the border to white again
jp .loop
ret
DATA1
defb 22,3,5 ; AT 3, 5
defb "Thank you"
DATA1END
Last edited by ParadigmShifter on Sat Oct 28, 2023 3:27 pm, edited 1 time in total.
Re: Short fallout style text game (Merged from multiple topics)
All text drawing is in assembly. It would be interesting to see how long it takes with the border though.
Re: Short fallout style text game (Merged from multiple topics)
yeah, using the border this way is common tech to measure timings on ZX. not only you can see how much of the frame time your code takes, but you can also see where exactly (well, almost exactly) CRT ray is at the given time. it really helps in writing arcade games where you're using "chase the ray" technique.
- ParadigmShifter
- Manic Miner
- Posts: 670
- Joined: Sat Sep 09, 2023 4:55 am
Re: Short fallout style text game (Merged from multiple topics)
I draw a little ruler on the right hand side of the frame if TIMING is set (only draw it once, before the main loop starts), so I can see exactly where the raster gets up to
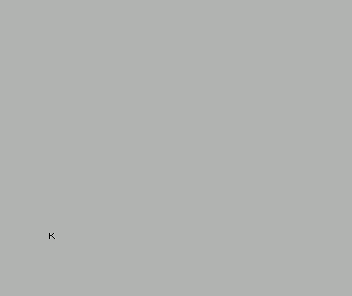
Black border: erasing the 6 16x16 sprites
Blue border: redrawing them. This finishes before the raster reaches top of the drawable area
Red border: populating clear list for next frame
Cyan border: drawing the debug collision minimap on the right
Magenta border: process keyboard update and do the collision and movement
Green border: populating draw list for next frame now I know where the pieces will be
Red border again: Animating the dotted lines around the piece preview (shows you where it will land)
White border: twiddling thumbs
The big delay when a piece is placed (still within a frame) is when the piece is placed and it is looking for 3 in a row (and updating the collision minimap again)
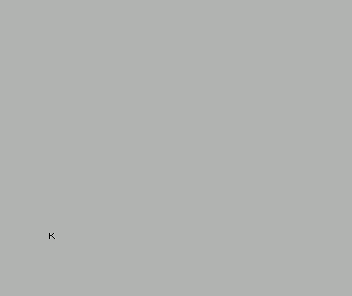
Black border: erasing the 6 16x16 sprites
Blue border: redrawing them. This finishes before the raster reaches top of the drawable area
Red border: populating clear list for next frame
Cyan border: drawing the debug collision minimap on the right
Magenta border: process keyboard update and do the collision and movement
Green border: populating draw list for next frame now I know where the pieces will be
Red border again: Animating the dotted lines around the piece preview (shows you where it will land)
White border: twiddling thumbs
The big delay when a piece is placed (still within a frame) is when the piece is placed and it is looking for 3 in a row (and updating the collision minimap again)
Re: Short fallout style text game (Merged from multiple topics)
I just thought, I only draw text once when something happens. So the border will appear for a split second. Hopefully I get to see it
- ParadigmShifter
- Manic Miner
- Posts: 670
- Joined: Sat Sep 09, 2023 4:55 am
Re: Short fallout style text game (Merged from multiple topics)
I usually just have a compile time switch so that if I want to I can do something every frame if I want to time it.
I use IF/ENDIF directives a lot in my code to enable debug code while I am writing it (so the border timing, the collision minimap, etc.). Then when I am satisfied it is fast as I can get it (or fast enough for now) I either turn it off or keep it on until I am ready to do a release build.
Some stuff I have an EQU for e.g. TIMING EQU 1 to turn it on and 0 to turn it off, other stuff which I know I don't want to turn on again once I have debugged and optimised it I just use IF 0 to turn it off (and if something goes wrong later on I can reenable the code with IF 1 again).
I use IF/ENDIF directives a lot in my code to enable debug code while I am writing it (so the border timing, the collision minimap, etc.). Then when I am satisfied it is fast as I can get it (or fast enough for now) I either turn it off or keep it on until I am ready to do a release build.
Some stuff I have an EQU for e.g. TIMING EQU 1 to turn it on and 0 to turn it off, other stuff which I know I don't want to turn on again once I have debugged and optimised it I just use IF 0 to turn it off (and if something goes wrong later on I can reenable the code with IF 1 again).
Re: Short fallout style text game (Merged from multiple topics)
Ah yes, that would be easy to do actually.
Just call the setup screen function every frame.
It does look like spectrum flicker on the gif so it probably is.
Just call the setup screen function every frame.
It does look like spectrum flicker on the gif so it probably is.
- ParadigmShifter
- Manic Miner
- Posts: 670
- Joined: Sat Sep 09, 2023 4:55 am
Re: Short fallout style text game (Merged from multiple topics)
If you are currently calling the ROM to clear the screen you might want to try one of these methods instead. Note - does not set the border colour
test code, just clears the screen in an endless loop
EDIT: Overlapping LDIR can be used for a fast(-ish) memset
memclr: just use value = 0
EDIT2: Need inc de instead of inc e, fixed. If address is even you can use inc e. Although...
EDIT3: The first macro can easily be made into a function instead (you'll have to dec bc yourself if you do that or pass length-1 instead). The second cannot because of the conditional assembly directives
You can also do a version which sets more than an 8 bit pattern e.g.
should set 16 bit values (untested)
Code: Select all
; A -> attrib to set when clearing screen
; at exit
; HL = 0
; B = 0
cls_fast:
di ;disable interrupt
ld (.stack+1), sp ;store current stack pointer
ld sp, 16384 + 6144 + 768
ld b, 128 ; clear attribs in 128 * 3 pushes
ld h, a
ld l, a
.attribloop
push hl
push hl
push hl
djnz .attribloop
ld hl, 0
.loop1
push hl
push hl
push hl
push hl
push hl
push hl
push hl
push hl
push hl
push hl
push hl
push hl
djnz .loop1
.stack
ld sp, 0 ;parameter will be overwritten
ei
ret
; A -> attrib to set when clearing screen
cls:
; set attribs
ld hl, #5800
ld de, #5801
ld bc, 768-1
ld (hl), a
ldir
; clear pixels
ld hl, #4000
ld (hl), b ; BC is 0 after above LDIR
ld de, #4001
ld bc, 6144-1
ldir
ret
test code, just clears the screen in an endless loop
Code: Select all
.clsagain
ld a, 2
out (#fe), a
ld a, 8+7; attrib to set (blue paper white ink)
IF 1 ; IF 1 : use stack abuse to set attribs and clear screen
call cls_fast
ELSE ; use overlapping LDIR/memset trick, code is small in size compared to fast version
call cls
ENDIF
ld a, 7
out (#fe), a
xor a
halt
jp .clsagain
Code: Select all
; do not use length of 1 otherwise bad things will happen
MACRO memset value, address, length
ld a, value
ld hl, address
ld d, h
ld e, l
inc de
ld bc, length - 1 ; this is why you can't use length of 1
ldir
ENDM
EDIT2: Need inc de instead of inc e, fixed. If address is even you can use inc e. Although...
Code: Select all
; now does correct thing if length is 1
MACRO memset value, address, length
ld a, value
ld hl, address
IF LENGTH == 1
ld (hl), a
ELSE
ld d, h
ld e, l
IF (address & 1 == 1)
inc de
ELSE
inc e
ENDIF
ld bc, length - 1 ; this is why you can't use length of 1
ldir
ENDIF
ENDM
You can also do a version which sets more than an 8 bit pattern e.g.
Code: Select all
ld bc, #0102; example 16 bit data to fill addresses with
ld hl, address
ld (hl), c ; low byte first
inc hl
ld (hl), b
ld d, h
ld e, l
inc de ; point DE to next 16 bits
dec hl ; point HL to first byte of data
ld bc, length - 2
ldir